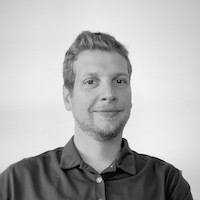
Fixinator's New Compatibility Scanner
Upgrading to the latest version of ColdFusion can be stressful.
From: Pete Freitag's Homepage
Upgrading to the latest version of ColdFusion can be stressful.
From: Pete Freitag's Homepage
Upgrading to the latest version of ColdFusion can be stressful.
From: Pete Freitag's Homepage
Upgrading to the latest version of ColdFusion can be stressful.
From: Pete Freitag's Homepage
This year at the Adobe ColdFusion summit in Las Vegas I presented on 20 ways to secure ColdFusion.
From: Pete Freitag's Homepage
This year at the Adobe ColdFusion summit in Las Vegas I presented on 20 ways to secure ColdFusion.
From: Pete Freitag's Homepage
This year at the Adobe ColdFusion summit in Las Vegas I presented on 20 ways to secure ColdFusion.
From: Pete Freitag's Homepage
I am going to attempt to keep this page updated with the latest ColdFusion Security Updates and Hotfixes published by Adobe.
From: Pete Freitag's Homepage
Links & Resources APSB25-15 - Adobe Product Security Bulletin CF2025 Update 11 - Adobe KB article for ColdFusion 2025 Update 1 CF2023 Update 13 - Adobe KB article for ColdFusion 2023 Update 13 CF2021 Update 19 - Adobe KB article for ColdFusion 2021 Update 19 Forum Thread - Adobe ColdFusi...
From: Pete Freitag's Homepage
Links & Resources APSB25-15 - Adobe Product Security Bulletin CF2025 Update 2 - Adobe KB article for ColdFusion 2025 Update 2 CF2023 Update 14 - Adobe KB article for ColdFusion 2023 Update 14 CF2021 Update 20 - Adobe KB article for ColdFusion 2021 Update 20 Forum Thread - Adobe ColdFusio...
From: Pete Freitag's Homepage
8 ) { //strip https:// website = right(website, len(website)-8); } As long as you are doing that kind of check, you should not have any issue with unexpected change of behavior due to this change.
From: Pete Freitag's Homepage
8 ) { //strip https:// website = right(website, len(website)-8); } As long as you are doing that kind of check, you should not have any issue with unexpected change of behavior due to this change.
From: Pete Freitag's Homepage
8 ) { //strip https:// website = right(website, len(website)-8); } As long as you are doing that kind of check, you should not have any issue with unexpected change of behavior due to this change.
From: Pete Freitag's Homepage
Last week's Adobe ColdFusion security update disabled searchImplicitScopes by default.
From: Pete Freitag's Homepage
Last week's Adobe ColdFusion security update disabled searchImplicitScopes by default.
From: Pete Freitag's Homepage
Adobe has published a ColdFusion Security Hotfix APSB24-14 today which describes "a critical vulnerability that could lead to arbitrary file system read".
From: Pete Freitag's Homepage
Adobe has published a ColdFusion Security Hotfix APSB24-14 today which describes "a critical vulnerability that could lead to arbitrary file system read".
From: Pete Freitag's Homepage
A few months ago I was on a mission to remove some of the old broken links on my blog.
From: Pete Freitag's Homepage
A few months ago I was on a mission to remove some of the old broken links on my blog.
From: Pete Freitag's Homepage
Removing the Server Header as of IIS 10 (the version of IIS installed by default on Windows Server 2016, 2019 or 2022) is now much easier than it had been with prior versions of IIS.
From: Pete Freitag's Homepage
Removing the Server Header as of IIS 10 (the version of IIS installed by default on Windows Server 2016, 2019 or 2022) is now much easier than it had been with prior versions of IIS.
From: Pete Freitag's Homepage
When setting up a server for training purposes I wanted to create a self signed certificate for app1.
From: Pete Freitag's Homepage
When setting up a server for training purposes I wanted to create a self signed certificate for app1.
From: Pete Freitag's Homepage